Vaadin Lazy Loading Techniques
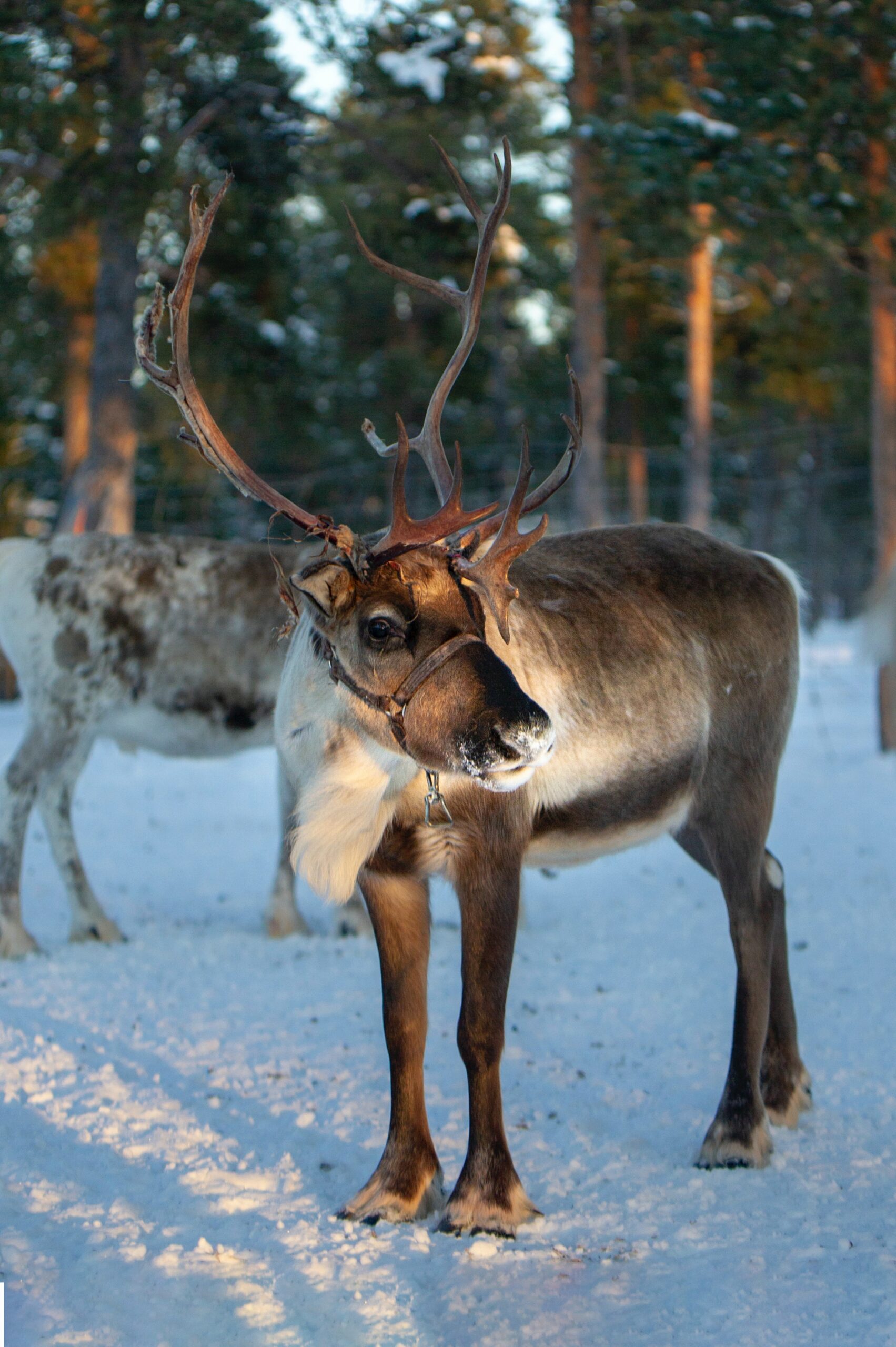
Working with massive datasets in Vaadin grids can cause poor performance. Lazy loading offers a powerful solution, allowing you to display data efficiently without overwhelming your application. This blog post dives into how to implement lazy loading in Vaadin using CallbackDataProvider.
Challenges of Large Datasets in Vaadin
When using grids, trees, or any other of the multi-valued component with Vaadin you often want to display data from a database table, and typically you have more than a few rows in the database.
In this case loading, thousands or even millions of records don’t make sense and would be a huge performance problem. For this use case, Vaadin provides lazy loading using a CallbackDataProvider
.
Vaadin Lazy Loading to the Rescue
To create a CallBackDataProvider
you must implement a CountCallback
and a FetchCallback
.
The CountCallback
is used to provide the total number of records. And the FetchCallback
is used for paging. Both methods receive an Query
object that contains filter, sorting, offset and limit.
In this example, you can see how to use offset and limit.
DataProvider<Employee, Void> dataProvider = new CallbackDataProvider<>( query -> employeeRepository.findAll(query.getOffset(), query.getLimit()), query -> employeeRepository.count() );
Ensuring Item Identity in Vaadin
In a Grid
or the DataProvider
there are methods that are using an item:
grid.select(employee); dataProvider.refreshItem(employee);
Ever wondered how Vaadin is finding the right item in the underlying data structure? No surprise – it uses equals()
.
But what if you can’t control how equals()
is implemented? For example, is the Class that you use in the Grid is generated directly from the database tables like jOOQ does?
No worries! Vaadin provides another constructor to create a CallbackDataProvivder
As a third parameter, you pass a ValueProvider that is responsible to return a unique identifier. In the example, this is the ID of the Employee.
DataProvider<Employee, Void> dataProvider = new CallbackDataProvider<>( query -> employeeRepository.findAll(query.getOffset(), query.getLimit()), query -> employeeRepository.count(), Employee::getId );
What’s next?
For even faster Vaadin grids, explore using editable functionality with our guide to Vaadin Editable Grid.
Looking to secure your Vaadin application? Check out our guide on building secure web apps with Vaadin and Spring Boot.
If you’re new to Vaadin, get started with the basics in our comprehensive guide: Beyond Angular and React: Building Web Apps with Vaadin.
- Hibernate Schema-based Multi-Tenancy using StatementInspector - 08.01.2024
- How do you get a Spring Bean without Dependency Injection? - 04.01.2024
- 2023 – What a Year! - 27.12.2023