Level Up Your Vaadin Apps with Vaadin Editable Grid
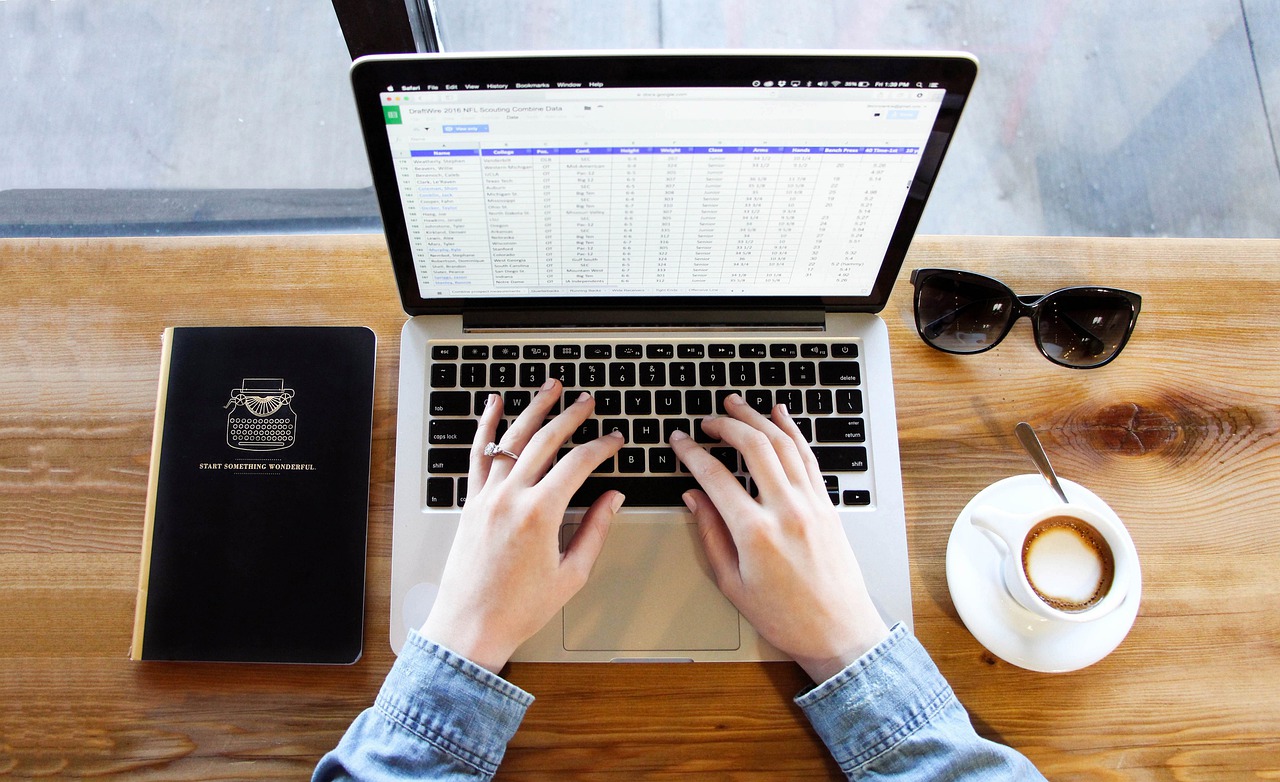
Vaadin has a fantastic Grid component that also can be made editable. But the configuration of the behavior, especially if you want to use the UI with the keyboard, can be complicated. In this blog post, I show you how this can be achieved.
The source code can be found on GitHub: https://github.com/simasch/vaadin-editable-grid
Demystifying the Vaadin Grid Editor
The example is based on the MasterDetailView that you get when creating a new project on https://start.vaadin.com
To make the Grid editable we must create a binder, get the editor from the grid and assign the binder.
var grid = new Grid<>(SamplePerson.class, false); var binder = new BeanValidationBinder<>(SamplePerson.class); // Create Grid Editor var editor = grid.getEditor(); editor.setBinder(binder); editor.setBuffered(true);
The grid editor has two modes: buffered and unbuffered. Buffered means changes must be explicitly committed, while unbuffered automatically commits changes on blur (when a field loses focus). In the example, the unbuffered mode is used as we explicitly want to save the editor and, in the save event, commit the changes to the database.
// Save Listener to save the changed SamplePerson editor.addSaveListener(event -> { SamplePerson item = event.getItem(); samplePersonService.update(item); });
Crafting Input Fields
Next, we must create the grid columns and assign an input component bound to the binder. Below is an example of one column with a text field.
var colFirstName = grid.addColumn("firstName"); var txtFirstName = new TextField(); binder.forField(txtFirstName).bind("firstName"); colFirstName.setEditorComponent(txtFirstName);
Grid Behavior – the Tricky Part
In the screenshot bellow, you can see how the grid looks in the edit mode. Now, how and when do we set the grid to edit mode?

One way to start editing is to simply select a row in the grid. Therefore we add a selection listener.
grid.addSelectionListener(event -> event.getFirstSelectedItem().ifPresent(samplePerson -> { editor.save(); if (!editor.isOpen()) { grid.getEditor().editItem(samplePerson); currentColumn.ifPresent(column -> { if (column.getEditorComponent() instanceof Focusable<?> focusable) { focusable.focus(); } } }));
When the row is clicked we first call save on the editor. If there is an open editor it will first validate with the binder and if the data is valid, write the changed values to the bean. If this works, the editor is closed, and a save event is sent. The save event is consumed by the save listener that was already introduced.
If the editor is closed after saving we pass the selected item to the editor to start editing. Now comes the tricky part: we want to focus the component in the column where the user clicked. To do that, we must know the current column. This has been done with a cell focus listener. There we not only store the current column but also the current item that we will use later.
grid.addCellFocusListener(event -> { // Store the item on cell focus. Used in the ENTER ShortcutListener currentItem = event.getItem(); // Store the current column. Used in the SelectionListener to focus the editor component currentColumn = event.getColumn(); });
As a convenience, we also want to provide keyboard navigation in the grid. Selecting a row is done by default with the spacebar, but we also want to support hitting the enter key. For that purpose, we register a shortcut. And in that shortcut, we use the previously saved current item.
Shortcuts.addShortcutListener(grid, event -> currentItem.ifPresent(grid::select), Key.ENTER).listenOn(grid);
Finally, we want to be able to cancel editing. Also there, we use a shortcut for the escape key:
Shortcuts.addShortcutListener(grid, () -> { if (editor.isOpen()) { editor.cancel(); } }, Key.ESCAPE).listenOn(grid);
Conclusion: Mastering Editable Vaadin Grids
Making a grid editable with Vaadin is straight forward if you know how to use the grid editor. If you want to add more convenience register the right shortcuts to make the user experience smoother.
I would like to thank Johannes from the support team for the tip with the cell focus listener that made this user experience improvement possible in the first place.
Ready to implement user-friendly editable grids in your Vaadin project? Get in touch if you need help with Vaadin editable grids!
Related Posts:
- Vaadin Keycloak OAuth2 Integration
- Vaadin Tip: Lazy Loading and Item Identity
- How to Deploy a Vaadin Application to Google Cloud App Engine
- Hibernate Schema-based Multi-Tenancy using StatementInspector - 08.01.2024
- How do you get a Spring Bean without Dependency Injection? - 04.01.2024
- 2023 – What a Year! - 27.12.2023